AI Class 9 Python Practical Work – The CBSE has changed the previous textbook and the syllabus of Std. IX. The new notes are made based on the new syllabus and based on the New CBSE textbook. All the important Information are taken from the Artificial Intelligence Class IX Textbook Based on CBSE Board Pattern.
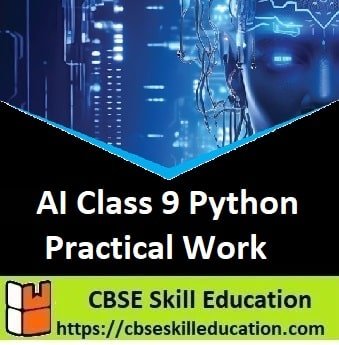
AI Class 9 Python Practical Work
Q. To print personal information like Name, Father’s Name, Class, School Name.
name = "Rakesh Kumar"
father_name = "Ajit Kumar"
class = "10th"
school_name = "XYZ High School"
print("Name: ", name)
print("Father's Name: ", father_name)
print("Class: ", class)
print("School Name: ", school_name)
Output:
Name: Rakesh Kumar
Father's Name: Ajit Kumar
Class: 10th
School Name: XYZ High School
Q. To print the following patterns using multiple print commands-
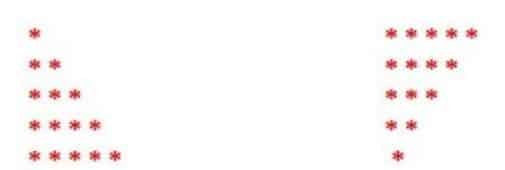
# Pattern A
print("*")
print("**")
print("***")
print("****")
print("*****")
Output:
*
**
***
****
*****
# Pattern B
print("*****")
print("****")
print("***")
print("**")
print("*")
Output:
*****
****
***
**
*
Q. To find square of number 7
number = 7
square = number ** 2
print("Square of", number, "is", square)
Output:
Square of 7 is 49
Q. To find the sum of two numbers 15 and 20.
num1 = 15
num2 = 20
sum_result = num1 + num2
print("Sum of", num1, "and", num2, "is", sum_result)
Output:
Sum of 15 and 20 is 35
Q. To convert length given in kilometers into meters.
kilometers = 5
meters = kilometers * 1000
print(kilometers, "kilometers is equal to", meters, "meters")
Output:
5 kilometers is equal to 5000 meters
Q. To print the table of 5 up to five terms.
number = 5
for i in range(1, 6):
print(number, "x", i, "=", number * i)
Output:
5 x 1 = 5
5 x 2 = 10
5 x 3 = 15
5 x 4 = 20
5 x 5 = 25
Q. To calculate Simple Interest if the principle_amount = 2000 rate_of_interest = 4.5 time = 10.
principal = 2000
rate = 4.5
time = 10
simple_interest = (principal * rate * time) / 100
print("Simple Interest is", simple_interest)
Output:
Simple Interest is 900.0
Q. To calculate Area and Perimeter of a rectangle
def rectangle(length, width):
area = length * width
perimeter = 2 * (length + width)
return area, perimeter
length = float(input("Enter the length of the rectangle: "))
width = float(input("Enter the width of the rectangle: "))
area, perimeter = rectangle(length, width)
print(f"Area: {area}, Perimeter: {perimeter}")
Output:
Enter the length of the rectangle: 25
Enter the width of the rectangle: 25
Area: 625.0, Perimeter: 100.0
Q. To calculate Area of a triangle with Base and Height
def triangle(base, height):
area = 0.5 * base * height
return area
base = float(input("Enter the base of the triangle: "))
height = float(input("Enter the height of the triangle: "))
area = triangle(base, height)
print(f"Area of the triangle: {area}")
Output:
Enter the base of the triangle: 25
Enter the height of the triangle: 25
Area of the triangle: 312.5
Q. To calculating average marks of 3 subjects
def average(subject1, subject2, subject3):
average = (subject1 + subject2 + subject3) / 3
return average
subject1 = float(input("Enter marks for subject 1: "))
subject2 = float(input("Enter marks for subject 2: "))
subject3 = float(input("Enter marks for subject 3: "))
average = average(subject1, subject2, subject3)
print(f"Average marks: {average}")
Output:
Enter marks for subject 1: 99
Enter marks for subject 2: 98
Enter marks for subject 3: 99
Average marks: 98.66666666666667
Q. To calculate discounted amount with discount %
price = float(input("Enter the original price: "))
d_percent = float(input("Enter the discount percentage: "))
d_amount = (d_percent / 100) * price
final_price = price - d_amount
print(f"Discount Amount: {d_amount:.2f}")
print(f"Final Price after Discount: {final_price:.2f}")
Output:
Enter the original price: 500
Enter the discount percentage: 10
Discount Amount: 50.00
Final Price after Discount: 450.00
Q. To calculate Surface Area and Volume of a Cuboid
length = float(input("Enter the length of the cuboid: "))
width = float(input("Enter the width of the cuboid: "))
height = float(input("Enter the height of the cuboid: "))
surface_area = 2 * (length * width + width * height + height * length)
volume = length * width * height
print(f"Surface Area of the Cuboid: {surface_area:.2f}")
print(f"Volume of the Cuboid: {volume:.2f}")
Output:
Enter the length of the cuboid: 7
Enter the width of the cuboid: 6
Enter the height of the cuboid: 2
Surface Area of the Cuboid: 136.00
Volume of the Cuboid: 84.00
Q. Create a list in Python of children selected for science quiz with following names- Arjun, Sonakshi, Vikram, Sandhya, Sonal, Isha, Kartik Perform the following tasks on the list in sequence-
- Print the whole list
- Delete the name “Vikram” from the list
- Add the name “Jay” at the end
- Remove the item which is at the second position.
children = ['Arjun', 'Sonakshi', 'Vikram', 'Sandhya', 'Sonal', 'Isha', 'Kartik']
print("Initial list:", children)
children.remove('Vikram')
print("After removing 'Vikram':", children)
children.append('Jay')
print("After adding 'Jay' at the end:", children)
children.pop(1)
print("After removing the second position item:", children)
Output:
Initial list: ['Arjun', 'Sonakshi', 'Vikram', 'Sandhya', 'Sonal', 'Isha', 'Kartik']
After removing 'Vikram': ['Arjun', 'Sonakshi', 'Sandhya', 'Sonal', 'Isha', 'Kartik']
After adding 'Jay' at the end: ['Arjun', 'Sonakshi', 'Sandhya', 'Sonal', 'Isha', 'Kartik', 'Jay']
After removing the second position item: ['Arjun', 'Sandhya', 'Sonal', 'Isha', 'Kartik', 'Jay']
Q. Create a list num=[23,12,5,9,65,44]
- print the length of the list
- print the elements from second to fourth position using positive indexing
- print the elements from position third to fifth using negative indexing
num = [23, 12, 5, 9, 65, 44]
print("Length of the list:", len(num))
print("Elements from second to fourth position:", num[1:4])
print("Elements from third to fifth position (negative indexing):", num[-4:-1])
Output:
Length of the list: 6
Elements from second to fourth position: [12, 5, 9]
Elements from third to fifth position (negative indexing): [5, 9, 65]
Q. Create a list of first 10 even numbers, add 1 to each list item and print the final list.
even_numbers = [x for x in range(2, 21, 2)]
final_list = [x + 1 for x in even_numbers]
print("Final list after adding 1 to each item:", final_list)
Output:
Final list after adding 1 to each item: [3, 5, 7, 9, 11, 13, 15, 17, 19, 21]
Q. Create a list List_1=[10,20,30,40]. Add the elements [14,15,12] using extend function. Now sort the final list in ascending order and print it.
List_1 = [10, 20, 30, 40]
List_1.extend([14, 15, 12])
List_1.sort()
print("Final sorted list:", List_1)
Output:
Final sorted list: [10, 12, 14, 15, 20, 30, 40]
Q. Program to check if a person can vote
age = int(input("Enter your age: "))
if age >= 18:
print("You are eligible to vote.")
else:
print("You are not eligible to vote.")
Output:
Enter your age: 19
You are eligible to vote.
Q. To check the grade of a student
marks = int(input("Enter your marks: "))
if marks >= 90:
grade = 'A'
elif marks >= 80:
grade = 'B'
elif marks >= 70:
grade = 'C'
elif marks >= 60:
grade = 'D'
else:
grade = 'F'
print("Your grade is:", grade)
Output:
Enter your marks: 98
Your grade is: A
Q. Input a number and check if the number is positive, negative or zero and display an appropriate message
number = float(input("Enter a number: "))
if number > 0:
print("The number is positive.")
elif number < 0:
print("The number is negative.")
else:
print("The number is zero.")
Output:
Enter a number: 8
The number is positive.
Q. To print first 10 natural numbers
for i in range(1, 11):
print(i)
Output:
1
2
3
4
5
6
7
8
9
10
Q. To print first 10 even numbers
for i in range(2, 21, 2):
print(i)
Output:
2
4
6
8
10
12
14
16
18
20
Q. To print odd numbers from 1 to n
n = int(input("Enter the value of n: "))
for i in range(1, n+1):
if i % 2 != 0:
print(i)
Output:
Enter the value of n: 6
1
3
5
Q. To print sum of first 10 natural numbers
sum = 0
for i in range(1, 11):
sum += i
print("The sum of the first 10 natural numbers is:", sum)
Output:
The sum of the first 10 natural numbers is: 55
Q. Program to find the sum of all numbers stored in a list
numbers = [int(x) for x in input("Enter numbers separated by space: ").split()]
sum = sum(numbers)
print("The sum of the numbers in the list is:", sum)
Output:
Enter numbers separated by space: 5 6
The sum of the numbers in the list is: 11
Updated Class 9 AI Notes
Subject Specific skills Notes (40 Marks)
- Unit 1: AI Reflection, Project Cycle and Ethics
- Unit 2: Data Literacy
- Unit 3: Math for AI (Statistics & Probability)
- Unit 4: Introduction to Generative AI
- Unit 5: Introduction to Python
Employability skills Notes ( 10 Marks )
- Unit 1 – Communication Skills
- Unit 2 – Self-Management Skills
- Unit 3 – Basic ICT Skills
- Unit 4 – Entrepreneurial Skills
- Unit 5 – Green Skills
Old Subject Specific skills Notes (For revision)
- Unit 1 – Introduction to Artificial Intelligence (AI)
- Unit 2 – AI Project Cycle
- Unit 3 – Neural Network
- Unit 4 – Introduction to Python
Disclaimer: We have taken an effort to provide you with the accurate handout of “AI Class 9 Python Practical Work“. If you feel that there is any error or mistake, please contact me at anuraganand2017@gmail.com. The above CBSE study material present on our websites is for education purpose, not our copyrights. All the above content and Screenshot are taken from Artificial Intelligence Class 9 CBSE Textbook and Support Material which is present in CBSEACADEMIC website, This Textbook and Support Material are legally copyright by Central Board of Secondary Education. We are only providing a medium and helping the students to improve the performances in the examination.
For more information, refer to the official CBSE textbooks available at cbseacademic.nic.in