Python Revision Tour Class 12 Notes helps to revisit the Python fundamental concepts covered in Class 12. Here is the summary of Python Revision Tour Class 12 – Complete Notes.
Introduction to Problem Solving
Introduction to problem-solving
Problem-solving is the process of identifying the issue, exploring the solutions, and implementing the most effective method to achieve the desired outcome. It is a method for overcoming the complex challenges in an effective method.
Steps for problem-solving
There are five different steps for solving the problem:
- Step 1: Analyzing the Problem: In this step, the problem is defined clearly.
- Step 2: Developing an Algorithm: Create a step-by-step plan to solve the problem.
- Step 3: Coding: Convert the algorithm to programming language to create an executable solution.
- Step 4: Testing: Check the code meets all the requirements and identify the issues.
- Step 5: Debugging: Resolve errors or bugs during the testing period and ensure the solution works correctly.
Representation of algorithms using flowcharts and pseudocode
A flowchart is a graphical or visual representation of an algorithm using various symbols, shapes, and arrows to explain a process or program, and pseudocode is a simple representation of an algorithm that uses the English language to describe coding logic.
An algorithm is a step-by-step process that provides a series of instructions that should be carried out in a particular order to get the desired outcome. Pseudocode and flowcharts are used to present algorithms.
Familiarization with the basics of Python programming
Introduction to Python – Python Revision Tour Class 12 Notes
Python is a popular high-level programming language for developing web-based applications, data science, and machine learning program.
Features of Python
Python is a simple and high-level programming language that has multiple features, like:
- Easy to learn
- Free and Open Source
- Object-oriented Language
- Cross-platform compatibility
Write a simple “hello world” program
Let’s see how we can write a simple Python program.
print("Hello, World!")
Python character set
The character set refers to the valid characters that are used in Python.
- Letters: a-z, A-Z
- Digits: 0-9
- Special Characters: +, -, *, /, =, <, >, etc.
- Whitespace: Space, tab, and newline (\n)
- Unicode Characters: Python supports Unicode from various languages.
Token in Python
The smallest unit in a Python program is called a token. In python all the instructions and statements in a program are built with tokens. Different type of tokens in python are keywords, identifier, Literals/values, operators and punctuators –
- Keywords: Words with a special meaning in a programming language are known as keywords. There are 33 keywords in Python True, False, class, break, continue, and, as, try, while, for, or, not, if, elif, print, etc.
- Identifiers: Identifier is a user-defined name given to a variable, function, class, module, etc. Python has certain guidelines for naming identifiers, follow these guidelines:
- Code in python is case – sensitive
- Always identifier starts with capital letter(A – Z), small letter (a – z) or an underscore( _ ).
- Digits are not allowed to define in first character, but you can use between.
- No whitespace or special characters are allowed.
- No keyword is allowed to define identifier.
- Literals: Literals are the raw data that is assigned to variables or constants during programming. There are five different types of literals string literals, numeric literals, Boolean literals and special literals none.
- String literals: The string literals in Python are represented by text enclosed in single, double, or triple quotations. Examples include “Computer Science,” ‘Computer Science’, ”’Computer Science”’ etc.
- Numeric Literals: Literals that have been used to storing numbers is known is Numeric Literals. There are basically three numerical literals – Integer, Float and Complex.
- Boolean Literal: Boolean literals have only two values True of False.
- Special literals none: The special literal “None” in Python used to signify no values, the absence of values, or nothingness.
- Operators: Operators are specialized symbols that perform arithmetic or logical operations. The operation in the variable is applied using operands. The operators can be:
- Arithmetic operators (+, -, * /, %, **, //)
- Bitwise operators (&, ^, |)
- Shift operators (<<, >>)
- Identity operators (is, is not)
- Relational operators (>, <, >=, <=, ==, !=)
- Logical operators (and, or)
- Assignment operator ( = )
- Membership operators (in, not in)
- Arithmetic-assignment operators (/=, +=, -=, %=, **=, //=).
- Punctuators: The structures, statements, and expressions in Python are organized using these symbols known as punctuators. Several punctuators are in python [ ] { } ( ) @ -= += *= //= **== = , etc.
What is variable?
A variable is just like a container which helps to contain the values. It serves as an object or element that uses memory space, which can contain a value, variable number, alphanumeric or both. Example, name = “Python”
Use of comments in python
The statement ignored by the Python interpreter during the execution is known as a comment. The comment starts with a hash symbol (#) in Python. It helps to add remarks in the source code.
Data types in Python
In Python, data types define the type of data that a variable can store. The following chart shows the different types of data types in Python.
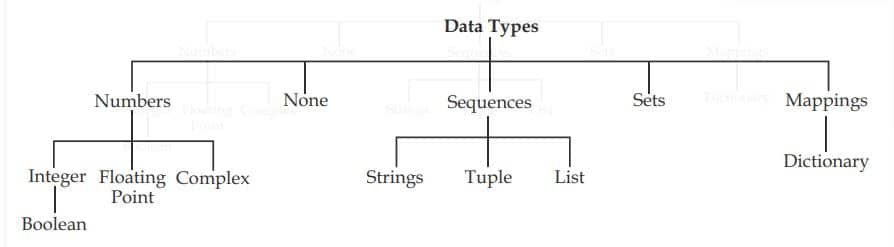

Operators in python
An operator is used to perform a specific mathematical or logical operation on values. The values that the operators work on are called operands. For example, in the expression 10 + num, the 10 is a value, the num is a variable, and the + (plus) sign is an operator.
- Arithmetic operators (+, -, * /, %, **, //)
- Bitwise operators (&, ^, |)
- Shift operators (<<, >>)
- Identity operators (is, is not)
- Relational operators (>, <, >=, <=, ==, !=)
- Logical operators (and, or)
- Assignment operator ( = )
- Membership operators (in, not in)
- Arithmetic-assignment operators (/=, +=, -=, %=, **=, //=).
Expressions in Python
Expressions are representations of value, An expression is defined as a combination of constants, variables, and operators. A value or a standalone variable is also considered as an expression but a standalone operator is not an expression. Some examples of valid expressions are:
Expressions | Expressions |
---|---|
100 | 3.0 + 3.14 |
num | 23/3 -5 * 7(14 -2) |
num – 20.4 | “Global” + “Citizen” |
Precedence of Operators
When an expression contains different kinds of operators, precedence determines which operator should be applied first. Higher precedence operator is evaluated before the lower precedence operator.
Precedence of all operators in Python
Precedence | Operators | Description |
---|---|---|
1 | ** | Exponentiation (raise to the power |
2 | ~ ,+, – | Complement, unary plus and unary minus |
3 | , / , % , // | Multiply, divide, modulo and floor division |
4 | + , – | Addition and subtraction |
5 | <= , < , > , >=, == , != | Relational and Comparison operators |
6 | =, %=, /=, //=, -=, +=,*=, **= | Assignment operators |
7 | is, is not | Identity operators |
8 | in, not in | Membership operators |
9 | not | Logical operators |
10 | and | Logical operators |
11 | or | Logical operators |
Statement in Python
In Python, a statement is a unit of code that the Python interpreter can execute.
>>> x = 4 #assignment statement
>>> cube = x ** 3 #assignment statement
>>> print (x, cube) #print statement
4 64
Input and output in Python
The Python program needs to interact with the user to get some input data or information. This is done by using the input() function, and the print() function helps to give output on the screen.
a. Input Function
fname = input("Enter your first name: ")
Enter your first name: Arnab
b. Output Function
fname = input("Enter your first name: ")
print(fname)
Enter your first name: Arnab
Arnab
Some more examples of Output function
Statement | Output |
---|---|
print(“Hello”) | Hello |
print(10 * 2.5) | 25.0 |
print(“I” + “love” + “my” + “country”) | Ilovemycountry |
print(“I’m”, 16, “years old”) | I’m 16 years old |
Type-conversion (explicit and implicit conversion)
Type conversion refers to converting one type of data to another type, and this data conversion can be done using two different ways:
- Explicit conversion: Explicit conversion done by the programmer manually using the cast operator.
- Implicit conversion: Implicit conversion is done automatically by the compiler; it is also known as coercion.
a. Explicit conversion example
num1 = 11
num2 = 2
print(num1/num2) #output: 5.5
print(int(num1/num2)) #output: 5
Function | Description |
---|---|
int(x) | Converts x to an integer |
float(x) | Converts x to a floating-point number |
str(x) | Converts x to a string representation |
chr(x) | Converts ASCII value of x to character |
ord(x) | returns the character associated with the ASCII code x |
b. Implicit conversion example
num1 = 10 #num1 is an integer
num2 = 20.0 #num2 is a float
sum1 = num1 + num2 #sum1 is sum of a float and an integer
print(sum1)
print(type(sum1))
Debugging in Python
A programmer can make mistakes while writing a program; the process of debugging, which helps to identify and remove mistakes, it is also known as bugs or errors. Errors are categorized as:
- Syntax errors: Every program has its own rules, and if a mistake is made in the program like missing any punctuation, incorrect command, or mismatched parentheses or braces, it can generate a syntax error.
- Logical errors: A logical error occurs when a program runs without errors but generates incorrect output, for example, assigning a value to the wrong variable, etc.
- Runtime errors: A runtime error occurs during the execution of a program or after the code is compiled or interpreted. For example, subtraction of two variables that hold string values, etc.
Statement Flow Control
Control flow refers to the sequence in which a program’s code is executed. Control flow statements manage the order in which code is executed and help to create a dynamic and flexible program.
Python has three types of control structures –
- Sequential – By default mode
- Selection – Used in decision making like if, switch etc.
- Repetition – It is used in looping or repeating a code multiple time
Conditional statements
The IF & IF-ELSE conditionals
The “IF-ELSE” conditionals help to check whether a condition is true or false; it is also known as a conditional statement. An “IF” statement executes a block of code only if the condition is true, while an “IF-ELSE” statement executes a block of code in both conditions, if the condition is true or false.
Syntax of IF condition –
if <conditional expression>:
[statement 1]
[statement 2]
Syntax of IF-ELSE condition –
if <conditional expression>:
[statement 1]
[statement 2]
else:
[statement 1]
[statement 2]
Nested IF statement
Nested if statements in Python allow the placing of one if statement inside another. Nested If is a powerful tool for building complex decision-making logic in your programs.
Syntax of Nested IF statement
if condition:
if condition:
[Statement]
else:
[Statement]
Looping Statement
In Python, looping statements are used to run a block of statements or code continuously for as many times as the user specifies. Python offers us two different forms of loops for loop and while loop.
The For loop
A “for” loop allows a block of code to be executed repeatedly until a condition is met. A for loop is used when you want to execute code multiple times or used for iterating over sequences like lists and arrays.
Syntax of FOR loop –
for <variable> in <sequence> :
statements_to_repeat
The range() based for loop
The range() function allows you to generate a sequence of numbers, which can be used in a for loop to iterate a specific number of times. It helps to control the flow of loops and can be used in several ways. By default range() function starts from 0.
Syntax –
range(stop)
range(start, stop)
range(start, stop, step)
The While loop
A while loop is a conditional loop that will repeat the instructions within itself as long as a conditional remain true.
Syntax –
while <logical expression> :
loop-body
Jump Statemement (break and condinue)
Python offers two jump statement – break and continue – to be used within loops to jump out of loop-iterations.
a. The break Statement
A break statement is used to terminate the loop based on the condition; a break loop is generally associated with an if statement. This loop termination can be used in a for loop, a do loop or a while loop.
Example:
a = b = c = 0
for i in range(1, 11) :
a = int(input("Enter number 1 :"))
b = int(input("Enter number 2 :))
if b == 0 :
print("Division by zero error! Aborting")
break
else
c=a/b
print("Quotient = ", c)
print("program over!")
b. The continue statement
Unlike break statement, the continue statement forces the next iteration of the loop to take place, skipping any code in between.
Syntax –
continue
Loop else statement
Python supports combining the else keyword with both the for and while loops. In a loop else statement, the else statement is used after the loop. The loop else statement will be executed once when the loop is completed without encountering a break statement.
Example,
for i in range(5):
print(i)
if i == 3:
break
else:
print("Loop completed without breaking.")
Nested Loops
A loop may contain another loop in its body. This form of a loop is called nested loop. But in a nested loop, the inner loop must terminate before the outer loop.
The following is an example of a nested loop :
for i in range(1,6) :
for j in range(1, i) :
print("*", end =' ')
print()
String in Python
The combination of characters is known as a string. A string is a fundamental data type in Python; it is immutable. Immutable means once a string is created, its value cannot be changed. Strings are enclosed in either single quotes (‘ ‘), double quotes (” “), or triple quotes (“”” “””). Triple quotes are basically used for multiple lines.
Basic Operation
Operation/ Method | Description | Example |
---|---|---|
Concatenation | Combine strings using + operator | “Hello ” + “World” (Output: Hello World) |
Repetition | Repeat strings using * operator | “Hi ” * 3 (Output: Hi Hi Hi) |
Membership | Check substring presence using in or not in | “a” in “apple” (Output: True) |
Slicing | Extract parts of the string using index ranges | “Hello”[0:4] (Output: Hell) |
String Method
Operation/ Method | Description | Example |
---|---|---|
len() | Returns length of the string | len(“Hello”) (Output: 5) |
capitalize() | Capitalizes first character | “hello”.capitalize() (Output: Hello) |
title() | Capitalizes first letter of each word | “hello world”.title() (Output: Hello World) |
lower() and upper() | Converts to lowercase/uppercase | “Hello”.lower() (Output: hello) |
count(substring) | Counts occurrences of a substring | “banana”.count(“a”) (Output: 3) |
find(substring) | Finds the first occurrence of substring | “banana”.find(“na”) (Output: 2) |
index(substring) | Like find() but raises error if not found | “banana”.index(“na”) (Output: 2) |
startswith()/ endswith() | Checks if string starts/ ends with a substring | “file.txt”.endswith(“.txt”) (Output: True) |
isalnum() | Checks if string is alphanumeric | “Python123”.isalnum() → True |
isalpha() | Checks if string is alphabetic | “Python”.isalpha() → True |
isdigit() | Checks if string is numeric | “12345”.isdigit() → True |
islower()/ isupper() | Checks if string is lowercase/ uppercase | “python”.islower() → True |
isspace() | Checks if string contains only whitespace | ” “.isspace() → True |
strip()/ lstrip()/ rstrip() | Removes whitespace | ” Hello “.strip() → Hello |
replace(old, new) | Replaces occurrences of a substring | “I like Java”.replace(“Java”, “Python”) |
join(iterable) | Joins elements of an iterable into a string | “, “.join([“a”, “b”]) → a, b |
partition(separator) | Splits into three parts (before, separator, after) | “hello”.partition(“l”) → (‘he’, ‘l’, ‘lo’) |
split(separator) | Splits string into a list of substrings | “a,b,c”.split(“,”) → [‘a’, ‘b’, ‘c’] |
List in Python
In Python, Multiple values (example, Number, Character, Date etc.) can be stored in a single variable by using lists., a list is an ordered sequence of elements that can be changed or modified. A list’s items are any elements or values that are contained within it. Lists are defined by having values inside square brackets [] just as strings are defined by characters inside quotations.
Example 1,
>>> list1 = [2,4,6,8,10,12]
>>> print(list1)
[2, 4, 6, 8, 10, 12]
Example 2,
>>> list2 = ['a','e','i','o','u']
>>> print(list2)
['a', 'e', 'i', 'o', 'u']
Example 3,
>>> list3 = [100,23.5,'Hello']
>>> print(list3)
[100, 23.5, 'Hello']
Tuples in Python
An ordered collection of components of various data kinds, such as integer, float, string, list, is known as a tuple. A tuple’s components are denoted by parenthesis (round brackets) and commas. A tuple’s elements can be retrieved using index values beginning at 0 just like list and string members can.
Example 1,
>>> tuple1 = (1,2,3,4,5)
>>> tuple1
(1, 2, 3, 4, 5)
Example 2,
>>> tuple2 =('Economics',87,'Accountancy',89.6)
>>> tuple2
('Economics', 87, 'Accountancy', 89.6)
Example 3,
>>> tuple3 = (10,20,30,[40,50])
>>> tuple3
(10, 20, 30, [40, 50])
Example 4,
>>> tuple4 = (1,2,3,4,5,(10,20))
>>> tuple4
(1, 2, 3, 4, 5, (10, 20))
Dictionary in Python
Maps include the data type dictionary. A collection of keys and a set of values are mapped in this situation. Items are keys and values pairs. Consecutive entries are separated by commas, and a colon (:) separates a key from its value. Dictionary entries are unordered, thus we might not receive the data in the same order that we entered it when we first placed it in the dictionary.
Example,
>>> dict3 = {'Mohan':95,'Ram':89,'Suhel':92,'Sangeeta':85}
>>> dict3
{'Mohan': 95, 'Ram': 89, 'Suhel': 92,'Sangeeta': 85}
FAQs on Python Revision Tour for Class 12 Students
What is Python Revision Tour in Class 12?
Python Revision Tour in Class 12 or Computational Thinking and Programming – 2 is a chapter of the CBSE Class 12 Computer Science syllabus that helps students revise essential Python concepts. It covers an introduction to Python, data types, operators, if statements, loops, functions, lists, strings, tuples, dictionaries and basic programs. It’s often included in Class 12 Computer Science notes and important question banks.
How to download Class 12 Python Revision Notes?
You can download the revision notes from the website using copy and paste or using a web browser.
What are the important topics in Python for Class 12 board exams?
The important topics in Python for class 12 are introduction to Python, data types, operators, if statements, loops, functions, lists, strings, tuples, dictionaries and basic programs.
Disclaimer: We have taken an effort to provide you with the accurate handout of “Python Revision Tour Class 12 Notes“. If you feel that there is any error or mistake, please contact me at anuraganand2017@gmail.com. The above CBSE study material present on our websites is for education purpose, not our copyrights. All the above content and Screenshot are taken from Computer Science Class 12 NCERT Textbook, CBSE Sample Paper, CBSE Old Sample Paper, CBSE Board Paper and CBSE Support Material which is present in CBSEACADEMIC website, NCERT websiteThis Textbook and Support Material are legally copyright by Central Board of Secondary Education. We are only providing a medium and helping the students to improve the performances in the examination.
Images and content shown above are the property of individual organizations and are used here for reference purposes only.
For more information, refer to the official CBSE textbooks available at cbseacademic.nic.in