Python in AI Class 11 Notes – The CBSE has updated the syllabus for St. XI (Code 843). The new notes are made based on the updated syllabus and based on the updated CBSE textbook. All the important information is taken from the Artificial Intelligence Class XI Textbook Based on the CBSE Board Pattern.
Python in AI Class 11 Notes
Python is a general-purpose, high level programming language. It was created by Guido van Rossum, and released in 1991. Python got its name from a BBC comedy series – “Monty Python’s Flying Circus”
Features of Python
- High Level language
- Interpreted Language
- Free and Open Source
- Platform Independent (Cross-Platform) – runs virtually in every platform if a
- compatible python interpreter is installed.
- Easy to use and learn – simple syntax similar to human language.
- Variety of Python Editors – Python IDLE, PyCharm, Anaconda, Spyder
- Python can process all characters of ASCII and UNICODE.
- Widely used in many different domains and industries.
Getting Started with Python Programs
Python program consists of Tokens. It is the smallest unit of a program that the interpreter or compiler recognizes. Tokens consist of keywords, identifiers, literals, operators, and punctuators. They serve as the building blocks of Python code.

a. Keywords: Reserved words used for special purpose. List of keywords are given below.

b. Identifier: An identifier is a name used to identify a variable, function, class, module or other object. Generally, keywords (list given above) are not used as variables. Identifiers cannot start with digit and also it can’t contain any special characters except underscore.
c. Literals: Literals are the raw data values that are explicitly specified in a program. Different types of Literals in Python are String Literal, Numeric Literal (Numbers), Boolean Literal (True & False), Special Literal (None) and Literal Collections.
d. Operators: Operators are symbols or keywords that perform operations on operands to produce a result. Python supports a wide range of operators:
- Arithmetic operators (+, -, *, /, %)
- Relational operators (==, !=, <, >, <=, >=)
- Assignment operators (=, +=, -=)
- Logical operators (and, or, not)
- Bitwise operators (&, |, ^, <<, >>)
- Identity operators (is, is not)
- Membership operators (in, not in)
e. Punctuators: Common punctuators in Python include: ( ) [ ] { } , ; . ` ‘ ‘ ” ” / \ & @ ! ? | ~ etc.
Data Types
Data types are the classification or categorization of data items. It represents the kind of value that tells what operations can be performed on a particular data. Python supports Dynamic Typing.
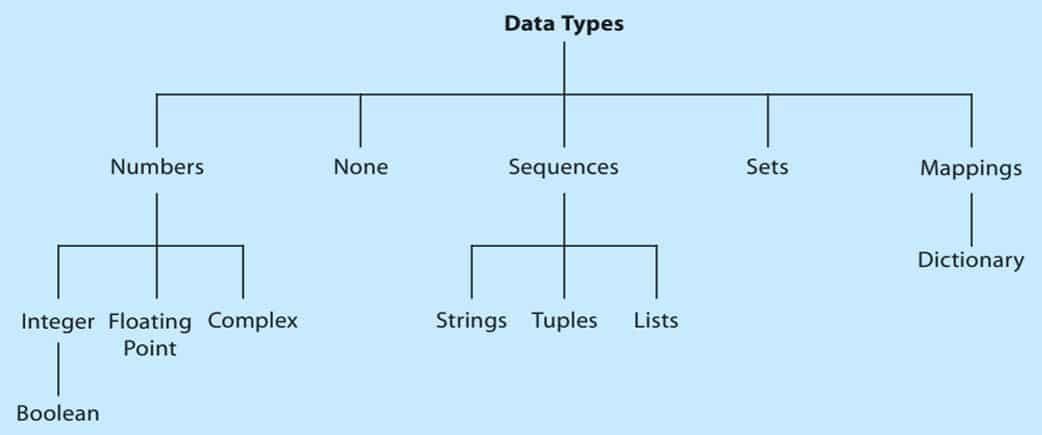
Data Type Description
Integer | Stores whole number | a=10 |
Boolean | Boolean is used to represent the truth values of the expressions. It has two values True & False | Result = True |
Floating point | Stores numbers with fractional part | x=5.5 |
Complex | Stores a number having real and imaginary part | num=a+bj |
String | Immutable sequences (After creation values cannot be changed in-place) Stores text enclosed in single or double quotes | name= “Ria” |
List | Mutable sequences (After creation values can be changed in-place) Stores list of comma separated values of any data type between square [ ] | lst=[ 25, 15.6, “car”, “XY”] |
Tuple | Immutable sequence (After creation values cannot be changed in-place) Stores list of comma separated values of any data type between parentheses ( ) | tup=(11, 12.3, “abc”) |
Set | Set is an unordered collection of values, of any type, with no duplicate entry. | s = { 25, 3, 3.5} |
Dictionary | Unordered set of comma-separated key:value pairs within braces {} | dict= { 1 : “One”, 2: “Two”, 3: “Three”} |
Accepting values from the user
The input() function retrieves text from the user by prompting them with a string argument. For instance:
name = input("What is your name?")
Sample Program,
Write a program to read name and marks of a student and display the total mark.
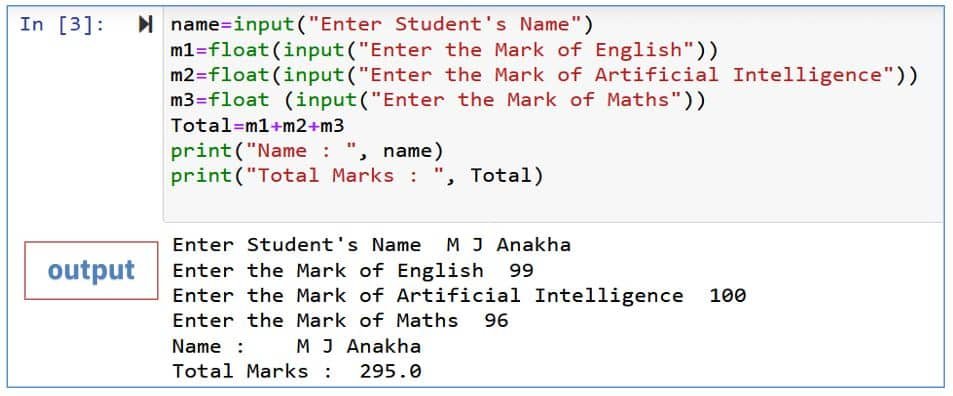
In the above example float( ) is used to convert the datatype into floating point. The explicit conversion of an operand to a specific type is called type casting.
Control flow statements in Python
Control flow is the order in which statements, instructions or functions are executed.

Selection Statement
The if/ if..else statement evaluates test expression and the statements written below will execute if the condition is true otherwise the statements below else will get executed. Indentation is used to separate the blocks.

Sample Program,
Asmita with her family went to a restaurant. Determine the choice of food according to the options she chooses from the main menu.

Looping Statements
Looping statements in programming languages allow you to execute a block of code repeatedly. In Python, there are mainly two types of looping statements: for loop and while loop.
For loop
The “for” keyword is used to start the loop. The loop variable takes on each value in the specified sequence (e.g., list, string, range). The colon (:) at the end of the for statement indicates the start of the loop body. The statements within the loop body are executed for each iteration.

The for loop iterates over each item in the sequence until it reaches the end of the sequence or until the loop is terminated using a break statement.
Simple program,
Q1. Write a program to display even numbers and their squares between 100 and 110.
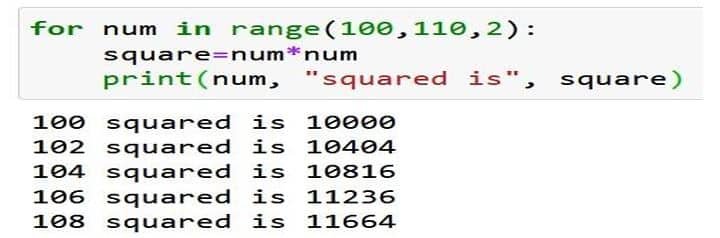
Q2. Write a program to read a list, display each element and its type. (use type( ) to display the data type.)

Q3. Write a program to read a string. Split the string into list of words and display each word.
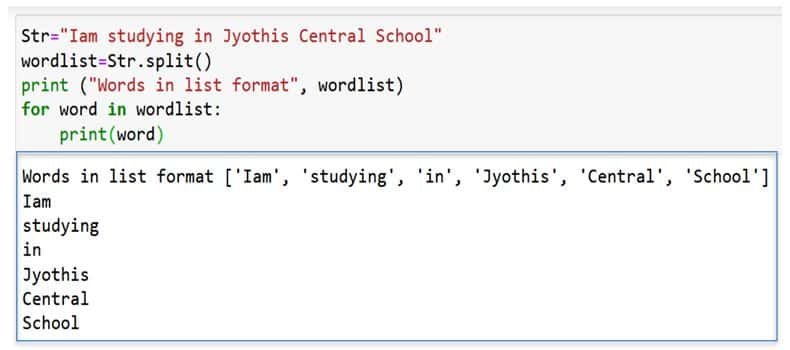
Q4. Write a simple program to display the values stored in dictionary
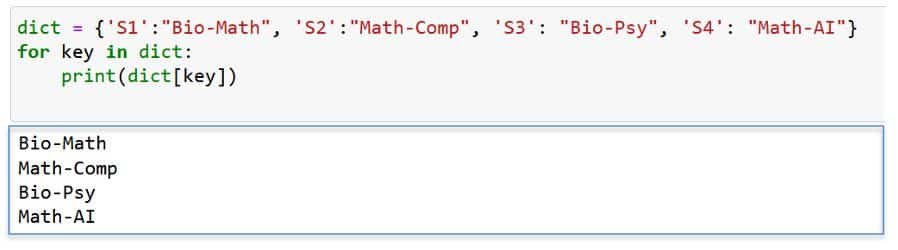
Understanding CSV file (Comma Separated Values)
CSV files are delimited files that store tabular data (data stored in rows and columns). It looks similar to spread sheets, but internally it is stored in a different format. In csv file, values are separated by comma. Data Sets used in AI programming are easily saved in csv format.
Let us see an example of opening, reading and writing formats for a file student.csv.
importing library | import csv |
Opening in reading mode | file= open(“student.csv”, “r”) |
Opening in writing mode | file= open(“student.csv”, “w”) |
closing a file | file.close( ) |
writing rows | wr=csv.writer(file) wr.writerow( [ 12, “Kalesh”, 480] ) |
Reading rows | details = csv.reader(file ) for rec in details: print(rec) |
Python program,
Write a Program to open a csv file students.csv and display its details

Introducing Libraries
In Python, functions are organized within libraries similar to how library books are arranged by subjects such as physics, computer science, and economics. For example, the “math” library contains numerous functions like sqrt(), pow(), abs(), and sin(), which facilitate mathematical operations and calculations.
For example, if we wish to use the sqrt() function in our program, we include the statement “import math”. This allows us to access and utilize the functionalities provided by the math library.
NUMPY
NumPy is also known as a numerical Python, is a powerful library in Python, which helps for numerical computing. NumPy is used for scientific computing and working with arrays.
Where and why do we use the NumPy library in Artificial Intelligence?
NumPy is a powerful tool for AI that provides multidimensional arrays and mathematical functions, which are critical in artificial intelligence. The NumPy library can also be used in data cleaning, model training, and the foundation of feature engineering.
NumPy can be installed using Python’s package manager, pip.

Creating a Numpy Array – Arrays in NumPy can be created by multiple ways. Some of the ways are programmed here:
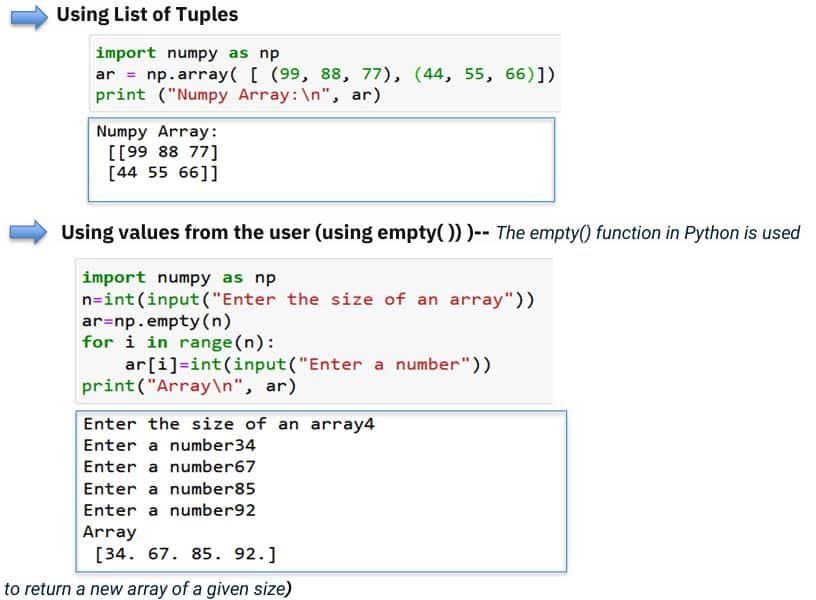
PANDAS
Pandas is an open-source Python library used for working with data sets. Pandas functions can analyze, explore, clean, and manipulate data. Pandas are used when we want to work on tabular data like CSV, Excel sheets, etc. The popularity of pandas in AI is for data analysis.
Where and why do we use the Pandas library in Artificial Intelligence?
Pandas provides powerful data manipulation and aggregation functionalities, making it easy to perform complex analysis and generate insightful visualizations. This capability is invaluable in AI and data-driven decision-making processes, allowing businesses to gain actionable insights from their data.
Series
A series is a one-dimensional labeled array that can hold any data types, like integers, floating numbers, strings, Python objects, etc. An index is the data label that corresponds to a specific value. For example,
Index | Data |
---|---|
0 | Rakesh |
1 | Ajit |
2 | Abheshk |
3 | Anil |
Two-dimensional datasets are commonly used in data science. Pandas DataFrames are useful in this situation. When we need to operate on several columns at once, we use a data frame.
Creation of DataFrame
There are several methods to create a DataFrame in Pandas, but here we will discuss two common approaches:


Dealing with Rows and Columns
A data frame is a two-dimensional data structure; data is arranged in tabular format in the form of rows and columns. To deal with rows and columns, some of the basic operations are required like adding new row or column, deleting row and column, accessing data frame element.
Adding a New Column to a DataFrame:
We can add a new column ‘Fathima’, by mentioning column name as given below
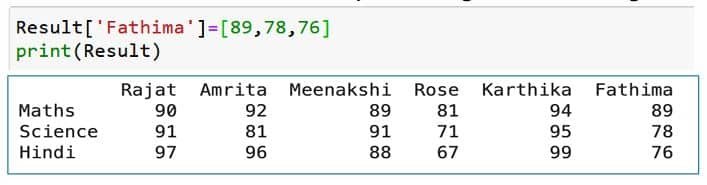
Adding a New Row to a DataFrame:
We can add a new row to a DataFrame using the DataFrame.loc[ ] method. Let us add marks for English subject in Result ➔
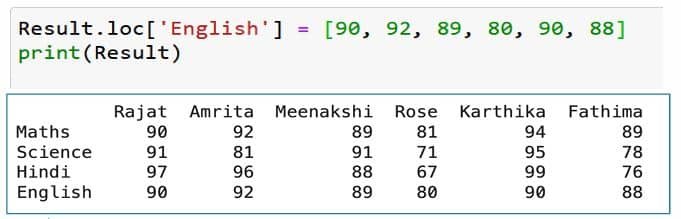
Deleting Rows and Columns from a DataFrame:
We need to specify the names of the labels to be dropped and the axis from which they need to be dropped.
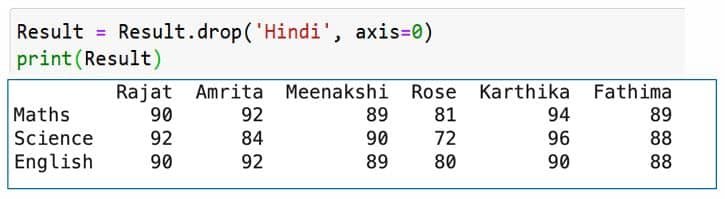
Delete the columns having labels ‘Rajat’, ‘Meenakshi’ and ‘Karthika’’:

Accessing DataFrame Elements
Data elements in a DataFrame can be accessed using different ways. Two common ways of accessing are using loc and iloc. DataFrame.loc[ ] uses label names for accessing and DataFrame.iloc[ ] uses the index position for accessing the elements of a DataFrame. Let us check an example

Understanding Missing Values
During Data Analysis, it is common for an object to have some missing attributes. If data is not collected properly it results in missing data. Pandas provide a function isnull() to check whether any value is missing or not in the DataFrame.

Attributes of DataFrames
Attributes are the properties of a DataFrame that can be used to fetch data or any information related to a particular DataFrame.
The syntax of writing an attribute is:

Let us understand the attributes of DataFrames with the help of DataFrame Teacher DataFrame:Teacher

Displaying Row Indexes – Teacher.index

Displaying column Indexes – Teacher.columns

Displaying datatype of each – Teacher.dtypes

Displaying data in Numpy Array form – Teacher.values

Displaying total number of rows and columns (row, column) – Teacher.shape

Displaying first n rows (here n = 2) – Teacher. head (2)
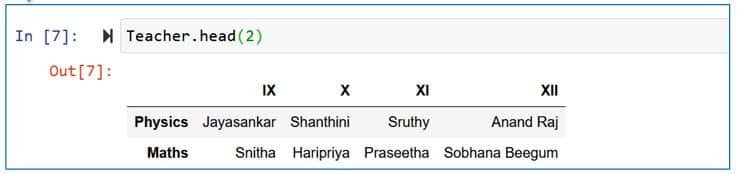
Displaying last n rows (here n = 2) – Teacher. tail (2)

Importing and Exporting Data between CSV Files and DataFrames
We can create a DataFrame by importing data from CSV files. Similarly, we can also store or export data in a DataFrame as a .csv file.
Importing a CSV file to a DataFrame
Using the read_csv() function, you can import tabular data from CSV files into pandas dataframe by specifying a parameter value for the file name.

Exporting a DataFrame to a CSV file
We can use the to_csv() function to save a DataFrame to a text or csv file. For example, to save the DataFrame Teacher into csv file resultout, we should write Teacher.to_csv(path_or_buf=’C:/PANDAS/resultout.csv’, sep=’,’)
Scikit-learn (Learn)
Scikit-learn (Sklearn) is the most useful and robust library for machine learning in Python. It provides a selection of efficient tools for machine learning and statistical modeling via a consistent interface in Python. Sklearn is built on (relies heavily on) NumPy, SciPy and Matplotlib.
Scikit-learn offers a variety of modules that simplify the process of building, training, and evaluating machine learning models, making it a popular choice for various tasks in this domain.
Key Features:
- Offers a wide range of supervised and unsupervised learning algorithms.
- Provides tools for model selection, evaluation, and validation.
- Supports various tasks such as classification, regression, clustering, dimensionality reduction, and more.
- Integrates seamlessly with other Python libraries like NumPy, SciPy, and Pandas.
load_iris (In sklearn.datasets): The Iris dataset is a classic and widely used dataset in machine learning, particularly for classification tasks.
train_test_split (In sklearn.model_selection): Datasets are usually split into training set and testing set. The training set is used to train the model and testing set is used to test the model. Most common splitting ratio is 80: 20. (Training -80%, Testing-20%)
KNeighborsClassifier (In sklearn.neighbors): Scikit-learn has wide range of Machine Learning (ML) algorithms which have a consistent interface for fitting, predicting accuracy, recall etc. Here we are going to use KNN (K nearest neighbors) classifier.
Disclaimer: We have taken an effort to provide you with the accurate handout of “Python in AI Class 11 Notes“. If you feel that there is any error or mistake, please contact me at anuraganand2017@gmail.com. The above CBSE study material present on our websites is for education purpose, not our copyrights. All the above content and Screenshot are taken from Artificial Intelligence Class 11 CBSE Textbook and Support Material which is present in CBSEACADEMIC website, This Textbook and Support Material are legally copyright by Central Board of Secondary Education. We are only providing a medium and helping the students to improve the performances in the examination.
Images shown above are the property of individual organizations and are used here for reference purposes only.
For more information, refer to the official CBSE textbooks available at cbseacademic.nic.in
1 thought on “Python in AI Class 11 Notes”